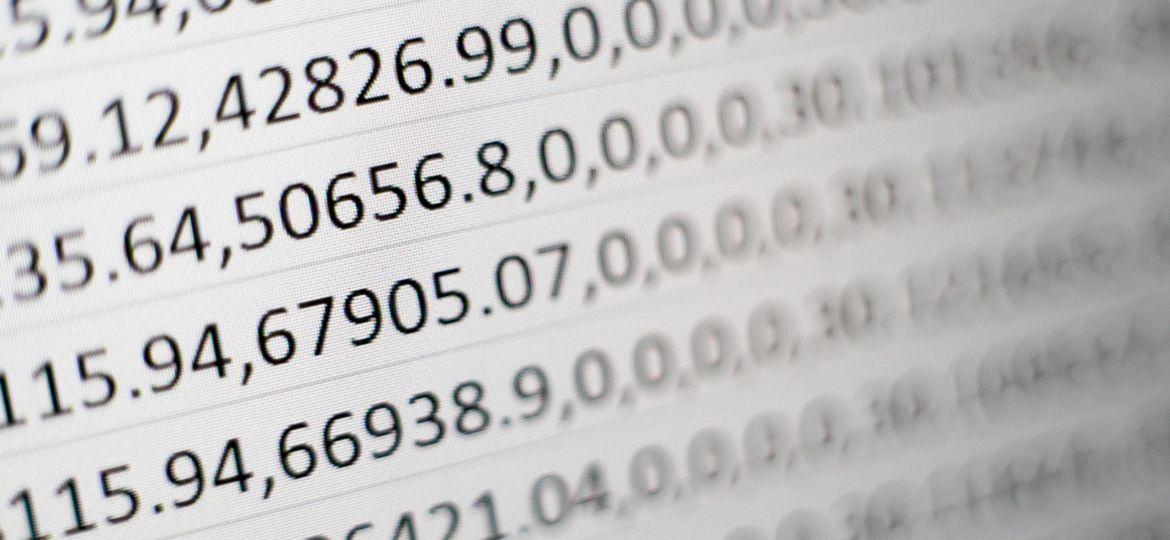
Simple API To Get Ethereum Supply And Data
Recently, there has been debate on Twitter on how to calculate the total supply of Ethereum?
In this article, we will showcase how to use are simple GraphQL APIs to get Ethereum supply and all sorts of other Ethereum blockchain data.
Before moving forward, a word about GraphQL.
GraphQL provides a complete and understandable description of the data in your API, to ask for precisely what data is needed.
Think of GraphQL as writing queries on a database through an API endpoint.
You can query more than 20 blockchains using Bitquery APIs, but today we will only talk about Ethereum.
Bitquery GraphQL endpoint — https://graphql.bitquery.io/
Yes, a single endpoint to get all sorts of blockchain data?
Ethereum total supply
To get Ethereum’s total supply, run the following query on Bitquery GraphQL IDE.
{
ethereum {
transactions {
gasValue
}
transfers(
currency: { is: "ETH" }
sender: { is: "0x0000000000000000000000000000000000000000" }
) {
amount
}
}
}
Here is an example of how to run above the GraphQL query?
As you can query result has two values, amount and gasValue. To get total Ethereum supply to subtract gasValue from the amount.
Total Ethereum supply = amount — gasValue
Total pre-mined Eth
More than 72 million ETH were initially pre-mined. To get the exact value of pre-mined ETH, use the following query.
{
ethereum {
transfers(
currency: { is: "ETH" }
height: { is: 0 }
sender: { is: "0x0000000000000000000000000000000000000000" }
receiver: { not: "0x0000000000000000000000000000000000000000" }
) {
amount
}
}
}
Ethereum pre-mine distribution
To know which addresses got the money in the Ethereum pre-mine, run the query below.
{
ethereum {
transfers(
currency: { is: "ETH" }
height: { is: 0 }
options: { desc: "amount" }
sender: { is: "0x0000000000000000000000000000000000000000" }
receiver: { not: "0x0000000000000000000000000000000000000000" }
) {
amount
receiver {
address
annotation
}
}
}
}
Total ETH sent to the genesis address
To know how many ETH were sent to the Ethereum genesis address, run the following query.
ETH or other tokens sent to genesis address is not recoverable.
{
ethereum {
burnt: transfers(
currency: { is: "ETH" }
receiver: { is: "0x0000000000000000000000000000000000000000" }
) {
amount
}
}
}
Large ( >100 ETH ) transfers to Ethereum genesis address
Run the query below to get the transactions sent to the Ethereum genesis address. The results will be in descending order of the transaction amount.
{
ethereum {
transfers(
currency: { is: "ETH" }
amount: { gt: 100 }
options: { desc: "amount" }
sender: { not: "0x0000000000000000000000000000000000000000" }
receiver: { is: "0x0000000000000000000000000000000000000000" }
) {
amount
transaction {
hash
}
block {
height
}
sender {
address
annotation
}
}
}
}
Total Gas spent on Ethereum.
Ethereum is an EVM, where any execution cost a fee, which is called Gas.
To calculate the total Gas spent on Ethereum, run the query below.
{
ethereum {
transactions {
gasValue
}
}
}
Total uncle blocks, transaction count and block reward
Uncles are stale blocks that contribute to the security of the main chain but are not considered the canonical “truth” for that particular chain height.
In contrast to orphan blocks (which have no parent), uncle blocks are linked to the chain but are not part of the final selection.
To get total uncle blocks, total transaction count, and total block rewards, run the following query.
{
ethereum {
blocks {
uncleCount
transactionCount
reward
}
}
}
Largest Ethereum transfers
Run the following query to get the largest Ethereum transactions. As you can see, the query is filtering transactions where more than 1 million ETH is transferred in a single transaction.
{
ethereum {
transfers(
currency: { is: "ETH" }
options: { desc: "amount", limit: 100 }
amount: { gt: 1000000 }
sender: { not: "0x0000000000000000000000000000000000000000" }
receiver: { not: "0x0000000000000000000000000000000000000000" }
) {
amount
transaction {
hash
}
sender {
address
annotation
}
receiver {
address
annotation
}
}
}
}
ETH emission and Gas spent for a given block
If you want to know how many Eth emitted and Gas spent for a given block, run the following query. In this query, we took block number 51 as an example.
{
ethereum {
transactions(height: { is: 51 }) {
gasValue
}
transfers(
height: { is: 51 }
currency: { is: "ETH" }
sender: { is: "0x0000000000000000000000000000000000000000" }
) {
amount
}
}
}
DAO fork transfers
DAO fork created Ethereum and Ethereum classic, therefore, to get the transactions that were included only in Ethereum during the fork, run the query below.
{
ethereum {
transfers(
height: { is: 1920000 }
currency: { is: "ETH" }
options: { desc: "amoun" }
receiver: { is: "0xbf4ed7b27f1d666546e30d74d50d173d20bca754" }
) {
amount
sender {
address
annotation
}
}
}
}
Ethereum transactions which used most Gas
Recently, there were two transactions(Tx1, Tx2) that used 10668 ETH Gas to transfer 350 ETH and 0.55 ETH.
Crazy! Right? Most probably, it was a mistake or coding error.
To know the Ethereum transactions that spent the largest gas amount, run the following query.
{
ethereum {
transactions(
options: { desc: "gasValue", limit: 10 }
gasValue: { gt: 100 }
) {
gasValue
sender {
address
annotation
}
hash
}
}
}
Get Ethereum token balance in a single API (Including NFTs)
Run the following query with parameters to get all Ethereum token balance in a single call. However, you need to subtract the sum_out from sum_in to get the actual balance.
balance = sum_in — sum_out
Query
query ($network: EthereumNetwork!, $address: String!) {
ethereum(network: $network) {
transfers(amount: { gt: 0 }, options: { desc: "count_in" }) {
sum_in: amount(calculate: sum, receiver: { is: $address })
sum_out: amount(calculate: sum, sender: { is: $address })
count_in: count(receiver: { is: $address })
count_out: count(sender: { is: $address })
currency {
symbol
}
}
}
}
Parameter
{
"network":"ethereum”,
"address":"0x2c82E14352e98c931852D9BB5f0760E36942CC3c"
}
Get Ethereum balance only
To get only the Ethereum balance of a given address, run the query below.
{
ethereum {
address(address: { is: "0x2c82E14352e98c931852D9BB5f0760E36942CC3c" }) {
balance
}
}
}
Bitquery Ethereum GraphQL APIs
Using our GraphQL APIs is similar to querying Ethereum blockchain directly.
You can get any data for Ethereum and other blockchains using Bitquery GraphQL APIs.
If you face any problem with the above queries or looking for any data, ping us on Telegram.
Also Read
- How to trace Bitcoin transactions or address?
- Blockchain Analytics: Everything you need to know
- Create a WhaleAlert like service in 10 minutes (Crypto Alert)
- gbwhatsapp télécharger
- Crypto chart widgets for your website (Including WordPress)
- Coinpath® – Blockchain Money Flow APIs
- Best Blockchain Analysis Tools and How They Work?
Subscribe to our newsletter
Subscribe and never miss any updates related to our APIs, new developments & latest news etc. Our newsletter is sent once a week on Monday.