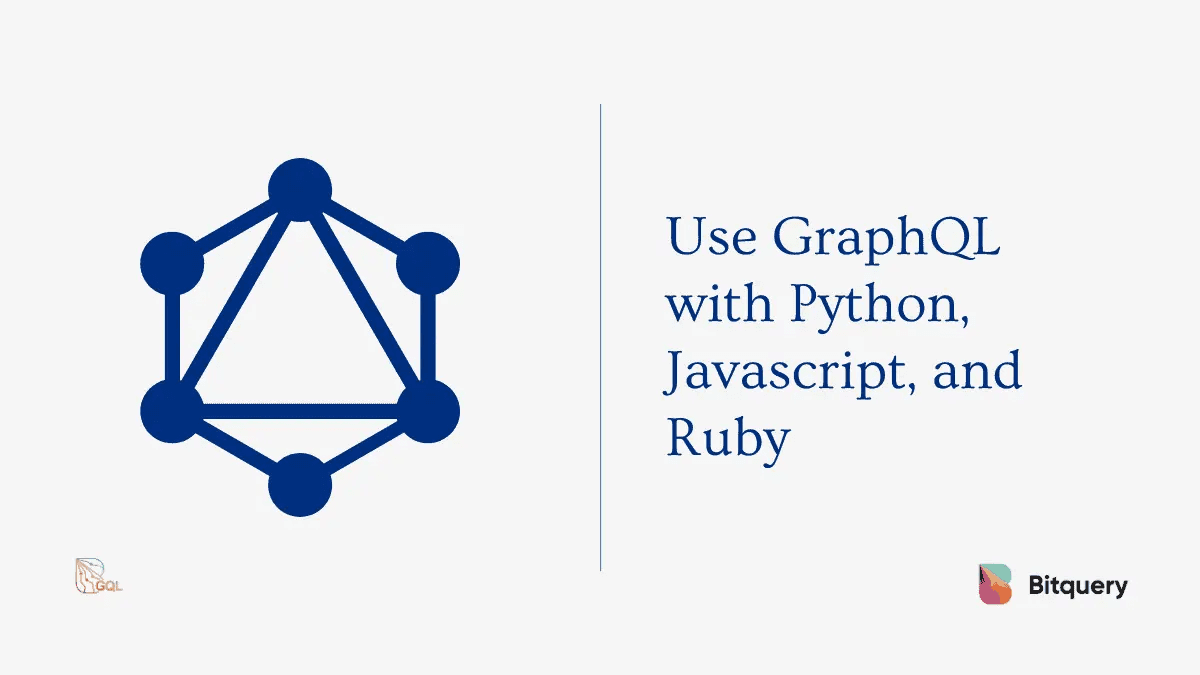
How to use GraphQL with Python, Javascript, and Ruby
In the last few articles, we talked argued using GraphQL APIs for blockchain data and how to create your first blockchain GraphQL query. In this article, we will show how to call GraphQL APIs using different programming languages.
However, you should always use our GraphQL playground to create and test your queries before embedding in your code.
Basics
Before starting, let’s cover some basics.
- All GraphQL requests are POST method.
- You also need API Key, which you get after signing up on our GraphQL IDE.
- The API key must be included as an HTTP header in every GraphQL request. The header name is X-API-KEY
- In GraphQL APIs endpoint remain the same for all the requests
- Our GraphQL endpoint is — https://graphql.bitquery.io/
We will be using the following GraphQL query in all our examples.
query{
bitcoin{
blocks{
count
}
}
}
Getting API Key
To get the API Key, sign up on our GraphQL IDE and then get the API key, which you can find under you account.
The API key must be included as an HTTP header in every GraphQL request. The header name is X-API-KEY
Now let’s see how to call GraphQL APIs using different programming languages.
GraphQL APIs using cURL
cURL allows using HTTP methods directly through our terminal. It is best for quick testing of any API request.
curl \\
-X POST \\
-H "Content-Type: application/json" \\
-H "X-API-KEY: YOU API KEY" \\
--data '{ "query": "{ bitcoin { blocks {count} } }" }' \\
https://graphql.bitquery.io/
GraphQL APIs using Python
Python is a beginner-friendly programming language. The example below shows how to call a GraphQL API using python.
#!/usr/bin/python
# -*- coding: utf-8 -*-
import requests
def run_query(query): # A simple function to use requests.post to make the API call.
headers = {'X-API-KEY': 'YOUR API KEY'}
request = requests.post('https://graphql.bitquery.io/',
json={'query': query}, headers=headers)
if request.status_code == 200:
return request.json()
else:
raise Exception('Query failed and return code is {}. {}'.format(request.status_code,
query))
# The GraphQL query
query = """
query{
bitcoin{
blocks{
count
}
}
}
"""
result = run_query(query) # Execute the query
print 'Result - {}'.format(result)
GraphQL Python Libraries
The libraries below are the most widely used libraries using GraphQL with python.
- Graphene — Graphene is an opinionated Python library for building GraphQL schemas/types fast and easily.
- Ariadne — Ariadne is a Python library for implementing GraphQL servers using a schema-first approach.
- Strawberry — Strawberry is a new GraphQL library for Python 3, inspired by data classes.
GraphQL APIs using Javascript
Javascript is one of the most adopted programming languages among developers all over the world. You can call a post request to get results from GraphQL APIs.
const query = `
query{
bitcoin{
blocks{
count
}
}
}
`;
const url = "https://graphql.bitquery.io/";
const opts = {
method: "POST",
headers: {
"Content-Type": "application/json",
"X-API-KEY": "YOUR API KEY"
},
body: JSON.stringify({
query
})
};
fetch(url, opts)
.then(res => res.json())
.then(console.log)
.catch(console.error);
GraphQL Javascript(NodeJS) Libraries
The following are the most popular javascript libraries for GraphQL.
- GraphQL.js — The JavaScript reference implementation for GraphQL, a query language for APIs created by Facebook.
- graphql-request — Minimal GraphQL client supporting Node and browsers for scripts or simple apps.
- Apollo client — Apollo Client is a fully-featured caching GraphQL client with integrations for React, Angular, and more. It allows you to build UI components that fetch data via GraphQL easily.
GraphQL APIs using Ruby
Ruby is also one of the most simple programming languages. The following example shows how to call a GraphQL API using ruby.
require 'net/http'
require 'uri'
require 'json'
uri = URI.parse("https://graphql.bitquery.io/")
header = {'Content-Type': 'application/json'; 'X-API-KEY': 'YOUR API KEY'}
query = "{ bitcoin { blocks { count } } }"
# Create the HTTP objects
http = Net::HTTP.new(uri.host, uri.port)
http.use_ssl = true
request = Net::HTTP::Post.new(uri.request_uri, header)
request.body = {query: query}.to_json
# Send the request
response = http.request(request)
puts "Body: #{response.body}"
GraphQL Ruby Libraries
You can use the libraries below to use GraphQL with ruby.
- graphql-ruby — Ruby implementation of Facebook’s GraphQL.
- graphql-client — A Ruby library for declaring, composing, and executing GraphQL queries.
- graphql-batch — A query batching executor for the graphql gem.
- agoo — Ruby web server that implements Facebook’s GraphQL.
- GQLi — A GraphQL client and DSL. Allowing to write queries in native Ruby.
Additional Resources
To see the examples of other programming languages, check the official website of GraphQL. In addition, to learn more about more GraphQL tools and libraries, check Awesome GraphQL.
If you have any questions or need help with your blockchain investigation, just hop on our Telegram channel. Also, let us know if you are looking for blockchain data APIs.
You might also be interested in:
- Create your first Blockchain GraphQL query
- API to get Ethereum Smart Contract Events
- Why GraphQL is better for blockchain data APIs
- APIs to get Latest Uniswap Pair Listing
- Simple rest APIs to get Uniswap data (DEX Data APIs)
- API to Get Ethereum Token Balance
- Simple API To Get Ethereum Supply And Data
- How to investigate an Ethereum address?
- Bitcoin Taproot – A Technical Explanation
- Who is actually using Ethereum?
- How to get newly created Ethereum Tokens?
- Querying Binance Smart Chain (BSC)
About Bitquery
Bitquery is a set of software tools that parse, index, access, search, and use information across blockchain networks in a unified way. Our products are:
-
Coinpath® APIs provide blockchain money flow analysis for more than 24 blockchains. With Coinpath’s APIs, you can monitor blockchain transactions, investigate crypto crimes such as bitcoin money laundering, and create crypto forensics tools. Read this to get started with Coinpath®.
-
Digital Assets API provides index information related to all major cryptocurrencies, coins, and tokens.
-
DEX API provides real-time deposits and transactions, trades, and other related data on different DEX protocols like Uniswap, Kyber Network, Airswap, Matching Network, etc.
If you have any questions about our products, ask them on our Telegram channel. Also, subscribe to our newsletter below, we will keep you updated with the latest in the cryptocurrency world.
Subscribe to our newsletter
Subscribe and never miss any updates related to our APIs, new developments & latest news etc. Our newsletter is sent once a week on Monday.